반응형
practice_blackjack.exe
11.04MB
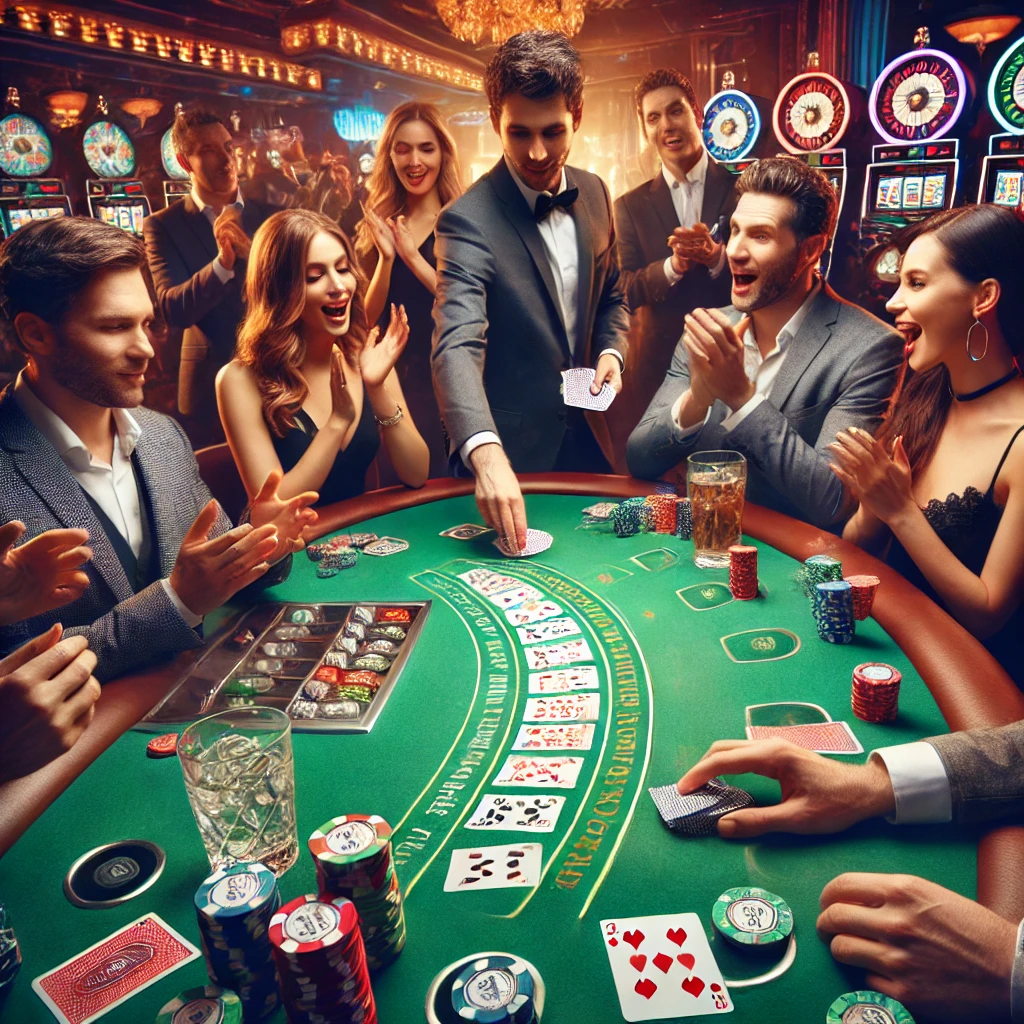
블랙잭 연습 프로그램과 게임 설명
블랙잭은 많은 사람들이 즐기는 카드 게임으로, 기본적인 규칙과 몇 가지 전략만 알면 누구나 쉽게 시작할 수 있습니다. 오늘은 블랙잭의 규칙과 전략에 대해 설명하고, 여러분이 직접 연습할 수 있는 간단한 블랙잭 게임 실행 프로그램을 제공하려 합니다.
블랙잭 기본 규칙
블랙잭의 목표는 딜러를 이기면서 카드의 합이 21을 넘지 않는 것입니다. 카드의 값은 다음과 같습니다:
- 숫자 카드(2~10): 표시된 숫자 그대로
- 얼굴 카드(잭, 퀸, 킹): 각 10점
- 에이스: 1점 또는 11점으로 플레이어가 선택
플레이어는 처음 두 장의 카드를 받은 후, 추가로 카드를 받을지(히트) 멈출지(스탠드)를 결정하게 됩니다. 딜러는 일반적으로 17점 이상에서 멈추고, 16점 이하에서 카드를 받습니다.
블랙잭 전략
더블다운 (Double Down)
더블다운은 처음 두 장의 카드 합이 유리할 때 베팅 금액을 두 배로 늘리고 한 장의 카드만 더 받는 전략입니다. 예를 들어, 처음 두 장의 카드 합이 11인 경우, 더블다운을 선택하면 높은 확률로 21에 가까워질 수 있습니다.
서렌더 (Surrender)
서렌더는 초기 두 장의 카드와 딜러의 첫 번째 카드 상황이 매우 불리할 때 사용합니다. 서렌더를 선언하면, 현재 베팅 금액의 절반을 돌려받고 게임에서 포기하게 됩니다. 서렌더는 모든 블랙잭 테이블에서 허용되지 않으므로, 게임을 시작하기 전에 확인이 필요합니다.
블랙잭 연습 프로그램
이제 간단한 블랙잭 게임을 연습할 수 있는 프로그램을 소개합니다. 이 프로그램은 Python으로 작성되었으며, 블랙잭의 기본 규칙을 따릅니다. 코드를 통해 게임의 흐름을 이해하고, 실제로 연습할 수 있습니다.
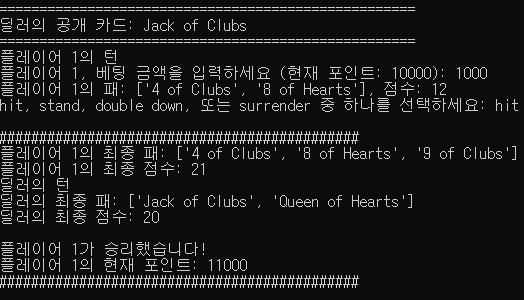
# 카드 덱 생성
def create_deck():
deck = []
for suit in ['Hearts', 'Diamonds', 'Clubs', 'Spades']:
for rank in range(2, 11):
deck.append(str(rank) + ' of ' + suit)
for face in ['Jack', 'Queen', 'King']:
deck.append(face + ' of ' + suit)
deck.append('Ace of ' + suit)
random.shuffle(deck)
return deck
# 카드의 값 계산
def calculate_hand_value(hand):
value = 0
num_aces = 0
for card in hand:
if card.startswith(('Jack', 'Queen', 'King')):
value += 10
elif card.startswith('Ace'):
num_aces += 1
value += 11
else:
value += int(card.split()[0])
while value > 21 and num_aces > 0:
value -= 10
num_aces -= 1
return value
# 블랙잭 게임 진행
def play_blackjack():
players = {
'플레이어 1': {'points': 10000},
# '플레이어 2': {'points': 10000},
# '플레이어 3': {'points': 10000}
}
while True:
deck = create_deck()
hands = {player: {'hand': [], 'bet': 0} for player in players}
hands['딜러'] = {'hand': [], 'bet': 0}
# 카드 2장씩 나누기
for player in hands:
hands[player]['hand'].append(deck.pop())
hands[player]['hand'].append(deck.pop())
# 딜러의 첫 번째 카드를 공개
print()
print('====================================================')
print(f"딜러의 공개 카드: {hands['딜러']['hand'][0]}")
print('====================================================')
# 플레이어의 턴 진행
for player in ['플레이어 1',
# '플레이어 2',
# '플레이어 3'
]:
if players[player]['points'] <= 0:
print(f"{player}는 더 이상 포인트가 없어 게임에서 제외되었습니다.")
continue
print(f"{player}의 턴")
bet = int(input(f"{player}, 베팅 금액을 입력하세요 (현재 포인트: {players[player]['points']}): "))
while bet > players[player]['points'] or bet <= 0:
bet = int(input(f"잘못된 베팅 금액입니다. {player}, 베팅 금액을 다시 입력하세요 (현재 포인트: {players[player]['points']}): "))
hands[player]['bet'] = bet
while True:
print(f"{player}의 패: {hands[player]['hand']}, 점수: {calculate_hand_value(hands[player]['hand'])}")
action = input("hit, stand, double down, 또는 surrender 중 하나를 선택하세요: ").strip().lower()
if action == 'hit':
hands[player]['hand'].append(deck.pop())
if calculate_hand_value(hands[player]['hand']) >= 21:
break
elif action == 'stand':
break
elif action == 'double down':
if len(hands[player]['hand']) == 2 and calculate_hand_value(hands[player]['hand']) in [9, 10, 11]:
hands[player]['bet'] *= 2
hands[player]['hand'].append(deck.pop())
break
else:
print("더블 다운은 두 장의 카드 합이 9, 10, 11일 때만 가능합니다.")
elif action == 'surrender':
if len(hands[player]['hand']) == 2:
players[player]['points'] -= hands[player]['bet'] // 2
hands[player]['bet'] = 0
print(f"{player}가 서렌더를 선택하여 베팅 금액의 절반을 잃습니다.")
break
else:
print("서렌더는 초기 두 장의 카드일 때만 가능합니다.")
else:
print("잘못된 선택입니다. 히트, 스탠드, 더블 다운, 또는 서렌더 중 하나를 선택하세요.")
print()
print('#############################################')
print(f"{player}의 최종 패: {hands[player]['hand']}")
print(f"{player}의 최종 점수: {calculate_hand_value(hands[player]['hand'])}")
# 딜러의 턴 진행
print("딜러의 턴")
while calculate_hand_value(hands['딜러']['hand']) < 17:
hands['딜러']['hand'].append(deck.pop())
print(f"딜러의 최종 패: {hands['딜러']['hand']}")
print(f"딜러의 최종 점수: {calculate_hand_value(hands['딜러']['hand'])}")
print()
# 결과 계산
dealer_value = calculate_hand_value(hands['딜러']['hand'])
for player in ['플레이어 1',
# '플레이어 2',
# '플레이어 3'
]:
if players[player]['points'] <= 0:
continue
player_value = calculate_hand_value(hands[player]['hand'])
if player_value > 21:
print(f"{player}가 버스트하여 패배했습니다.")
players[player]['points'] -= hands[player]['bet']
elif dealer_value > 21 or player_value > dealer_value:
print(f"{player}가 승리했습니다!")
players[player]['points'] += hands[player]['bet']
elif player_value < dealer_value:
print(f"{player}가 패배했습니다.")
players[player]['points'] -= hands[player]['bet']
else:
print(f"{player}가 딜러와 비겼습니다.")
print(f"{player}의 현재 포인트: {players[player]['points']}")
print('#############################################')
# 게임 종료 여부 확인
if all(players[player]['points'] <= 0 for player in [
'플레이어 1',
# '플레이어 2',
# '플레이어 3'
]):
print("모든 플레이어가 포인트를 다 잃었습니다. 게임 종료.")
break
if __name__ == '__main__':
play_blackjack()
반응형
'자동화' 카테고리의 다른 글
[202407] 파이썬 Selenium을 통한 크롤링 방법(스크롤 이동 포함) (4) | 2024.07.22 |
---|---|
[202407] 크롬 및 크롬드라이버 버전 114 설치 방법 (3) | 2024.07.22 |
[202407] 마우스 자동 반복 클릭 프로그램(다운로드) pw : 1993 (1) | 2024.07.18 |
[202407] 엑셀 파일 합치기 프로그램 [필터 제거, 컬럼 추출 포함](다운로드) (1) | 2024.07.17 |
[202407] PC 최적화 및 유지보수 자동화 툴(다운로드) (0) | 2024.07.15 |